Pionex Strategy Executor via Webhooks
Role: Lead Developer | Duration: September 2023
The Pionex Strategy Executor is an automated trading system that leverages webhooks to execute trading strategies on the Pionex cryptocurrency exchange. This solution provides seamless execution with real-time portfolio tracking and zero-fee transactions, including strategic profit analysis for informed investment decisions.
Experimental Project Disclaimer: This project was developed purely for experimental and educational purposes. The strategies and implementations demonstrated here are not financial advice. Cryptocurrency trading involves significant risks, and all trading decisions should be made based on your own research and risk tolerance. The project showcases technical implementation capabilities only.
Development Timeline
Research & Planning
Early September 2023
- API documentation analysis
- Strategy formulation
- Risk management planning
- System architecture design
Core Development
Mid September 2023
- Webhook endpoint setup
- API integration implementation
- Error handling system
- Testing framework creation
Strategy Implementation
Late September 2023
- Trading logic development
- Position management system
- Risk controls implementation
- Performance monitoring setup
Testing & Optimization
End of September 2023
- Live testing with small positions
- Strategy refinement
- Performance optimization
- Documentation completion
System Architecture
System Overview
Trading Signal Flow
Risk Management Process
Implementation Details
Webhook Handler
@app.post("/webhook")
async def webhook_handler(request: Request):
data = await request.json()
# Validate incoming signal
if not validate_signal(data):
logger.error("Invalid signal format")
return {"status": "error", "message": "Invalid signal format"}
# Process trading signal
try:
strategy = StrategyExecutor(data)
result = await strategy.execute()
return {"status": "success", "data": result}
except Exception as e:
logger.error(f"Strategy execution failed: {str(e)}")
return {"status": "error", "message": str(e)}
Risk Management Implementation
class RiskManager:
def __init__(self, config):
self.max_position_size = config.MAX_POSITION_SIZE
self.max_exposure = config.MAX_EXPOSURE
self.stop_loss_pct = config.STOP_LOSS_PCT
async def validate_trade(self, trade_params):
# Check account balance
balance = await self.get_account_balance()
if trade_params.size > self.max_position_size * balance:
raise RiskLimitExceeded("Position size exceeds maximum allowed")
# Check total exposure
current_exposure = await self.get_total_exposure()
if current_exposure + trade_params.size > self.max_exposure:
raise RiskLimitExceeded("Total exposure limit exceeded")
return True
Deployment Architecture
Server Setup
- Vultr Cloud Compute Instance
- Ubuntu 20.04 LTS
- 2 vCPU, 2GB RAM
- Automated backups
Security Measures
- SSL/TLS encryption
- UFW firewall configuration
- API key authentication
- Rate limiting
Process Management
- PM2 process manager
- Automatic restarts
- Load balancing
- Performance monitoring
Deployment Configuration
# Nginx Configuration
server {
listen 443 ssl;
server_name trading.example.com;
ssl_certificate /etc/letsencrypt/live/trading.example.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/trading.example.com/privkey.pem;
location / {
proxy_pass http://localhost:8000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
# PM2 Ecosystem Config
{
"apps": [{
"name": "trading-bot",
"script": "main.py",
"interpreter": "python3",
"instances": 2,
"exec_mode": "cluster",
"watch": true,
"max_memory_restart": "300M"
}]
}
Key Features
Real-time Execution
- Instant webhook processing
- Low-latency order execution
- Real-time status updates
Portfolio Tracking
- Live position monitoring
- Performance analytics
- Risk management
Risk Management
- Position size limits
- Stop-loss automation
- Exposure controls
Strategy Flexibility
- Custom strategy support
- Multiple timeframe analysis
- Parameter optimization
Performance Metrics
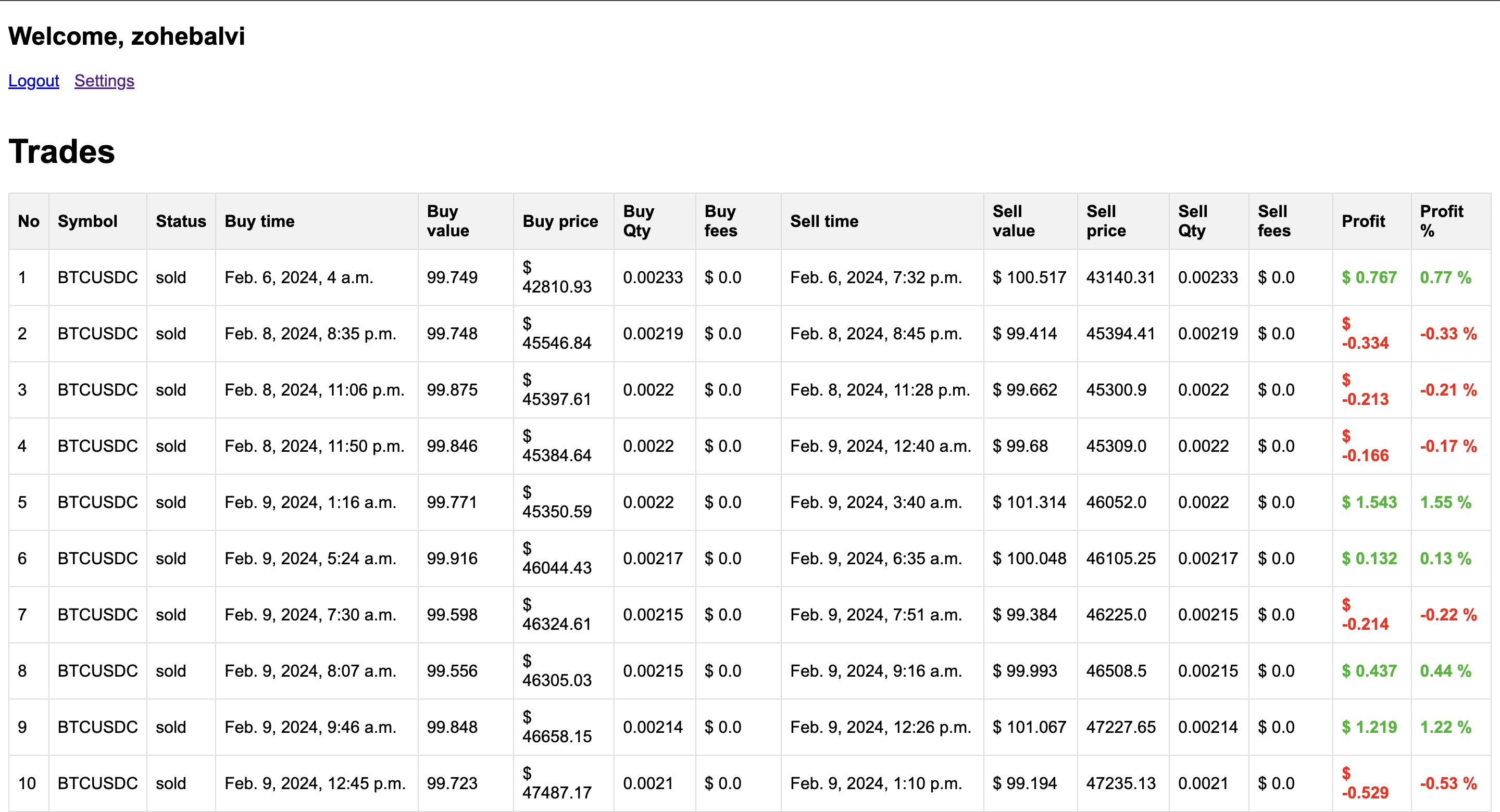
Live Trade Report Dashboard
Execution Speed
Average order execution time
Success Rate
Order execution accuracy
Win Rate
Strategy success rate
TradingView Integration
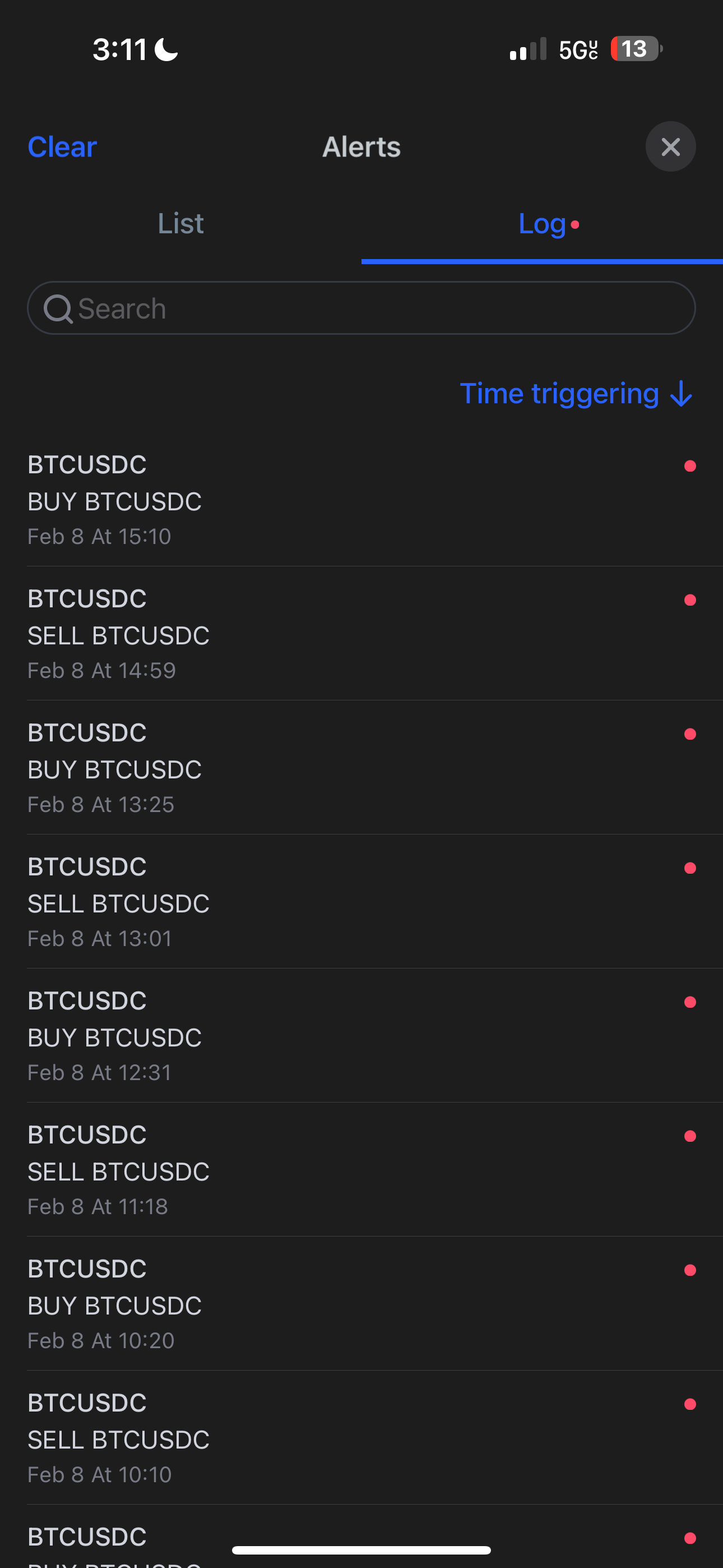
Alert Configuration
TradingView alert setup with webhook integration and custom message format.
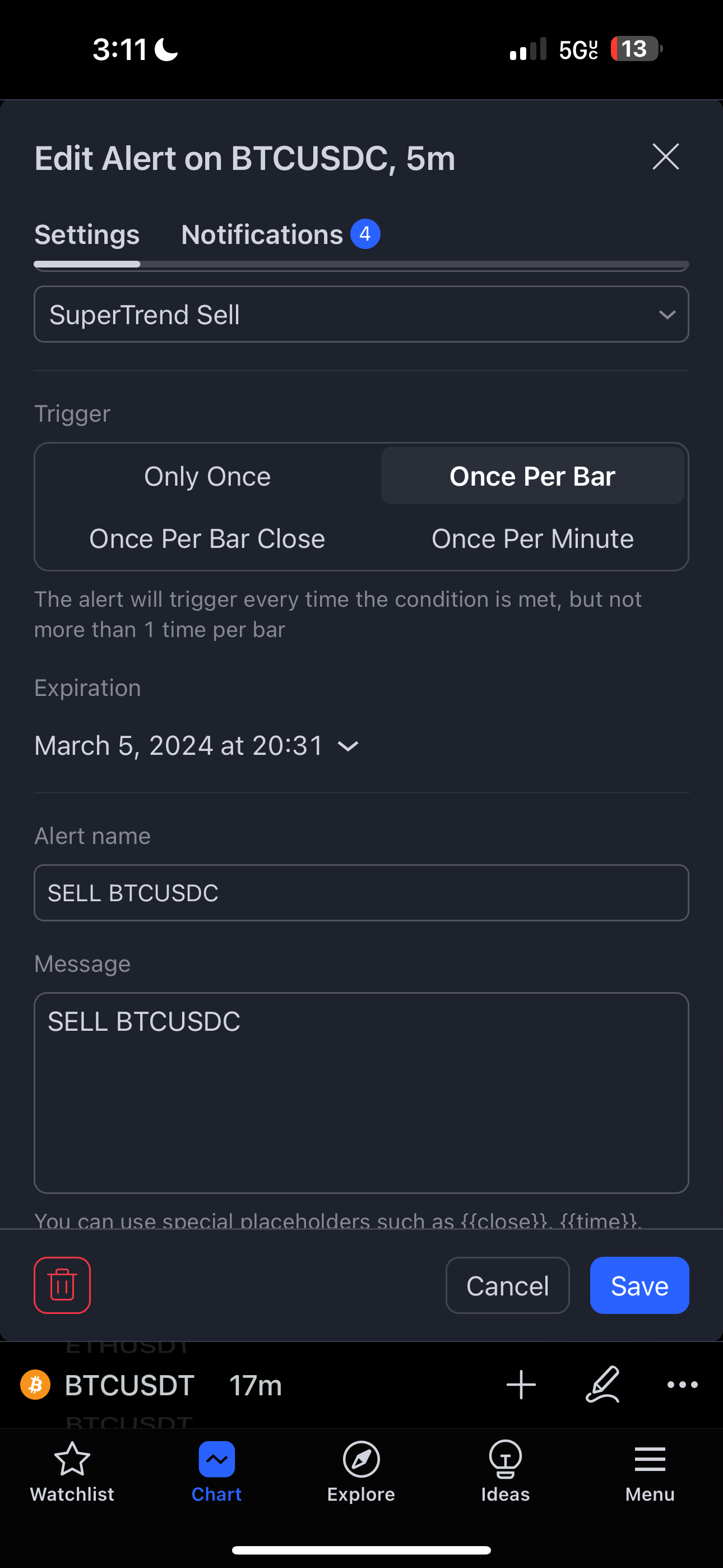
Strategy Implementation
Custom strategy implementation with technical indicators and signal generation.
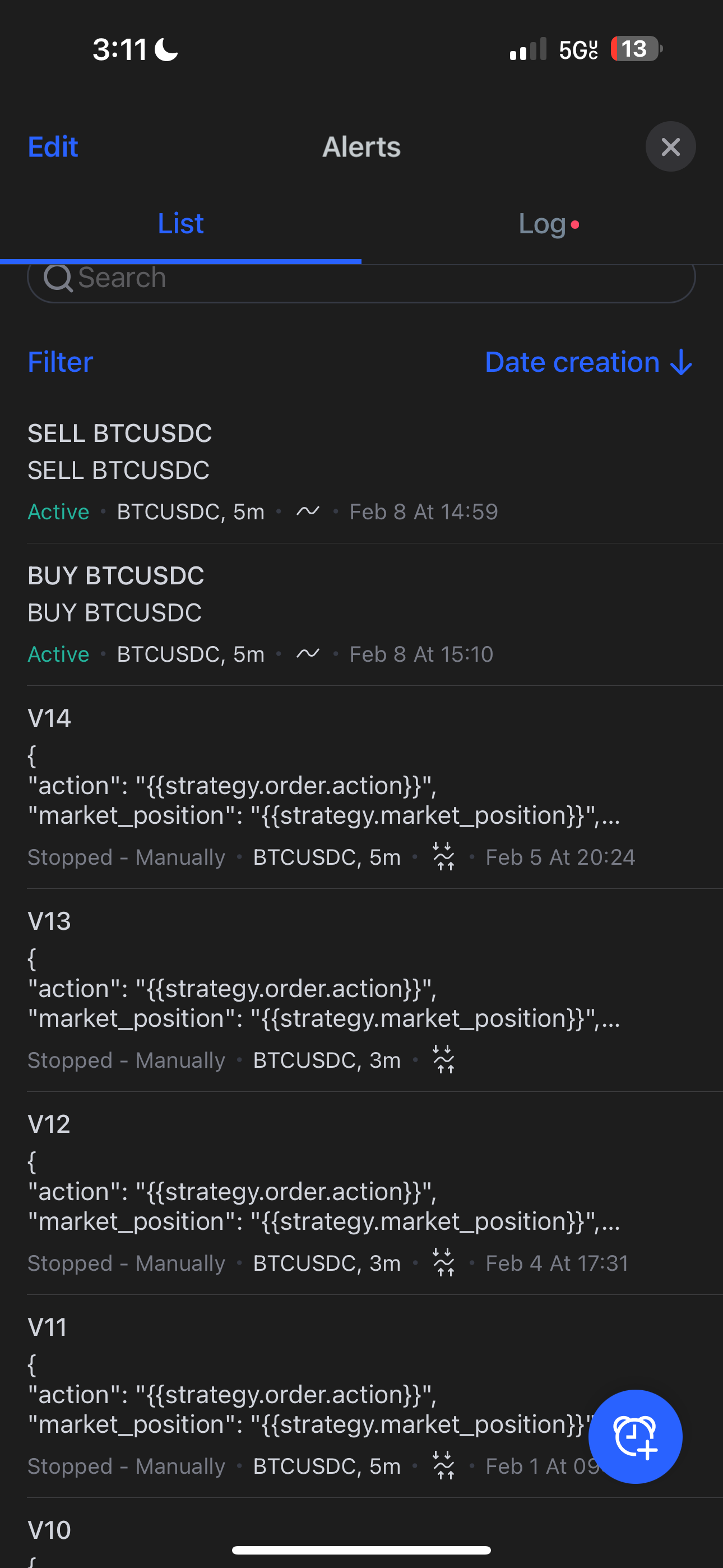
Performance Dashboard
Real-time monitoring of strategy performance and trade execution.
Project Resources
Access the project files and documentation for the Pionex Strategy Executor system.
Strategy Executor Package
Complete implementation including webhook handler, strategy executor, and documentation.